Verify SDK for iOS and Android
In this comprehensive guide, we'll navigate you through the essential steps to kickstart your journey with the Verify SDK on Mobile native apps.
iOS
Add package
pod 'SynapsVerify'
Usage
You must first Init a new session using your backend and fetch it using your private authenticated API. You will then need to replace: self.$sessionId by the initialized session ID.
...
@State private var sessionId = ""
var body: some View {
...
Synaps(sessionId: self.$sessionId,
primaryColor: .blue,
secondaryColor: .white, ready: {
print("ready")
}, finished: {
print("finished")
})
...
Properties
- Name
sessionId
- Type
- string
- Description
Unique verification session identifier
- Name
type
- Type
- string
- Description
individual for KYC (default)
corporate for KYB
- Name
ready()
- Type
- callback
- Description
Called when SDK is ready
- Name
finished()
- Type
- callback
- Description
Called when user finishes the verification process
- Name
lang
- Type
- string (optional)
- Description
User language preference
en - English
fr - French
de - German
es - Spanish
it - Italian
ja - Japanese
ko - Korean
pt - Portuguese
ro - Romanian
ru - Russian
tr - Turkish
vi - Vietnamese
zh-CN - Simplified Chinese
zh-TW - Traditional Chinese
- Name
tier
- Type
- string (optional)
- Description
Tier ID
2. StoryBoard
How to setup it
The library allows you to use all the features of standard View with a lot of new cool features, customizable from Storyboard or from code.
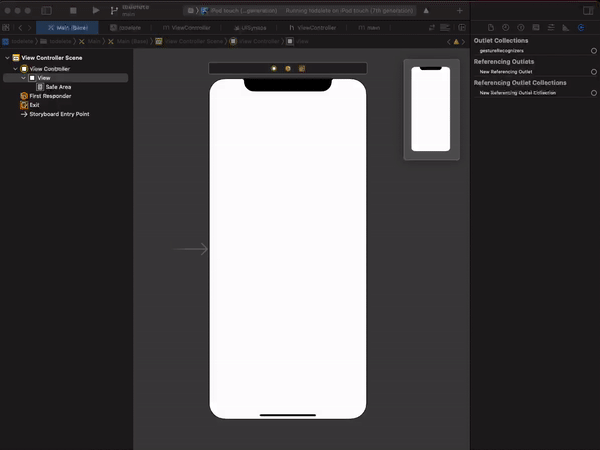
First of all, drag & drop a new View inside your view controller in storyboard.
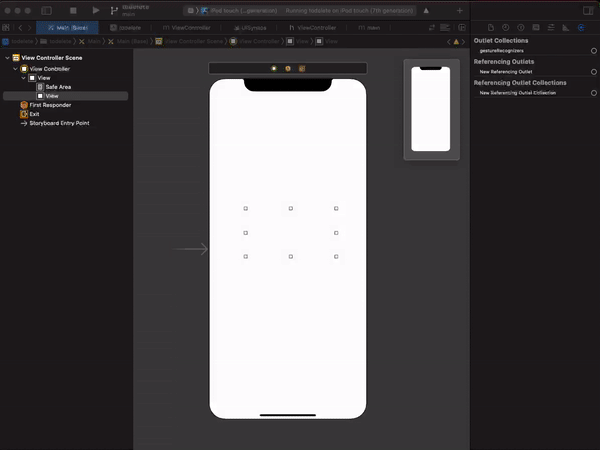
Then set the View class to UISynapsIndividual or UISynapsCorporate
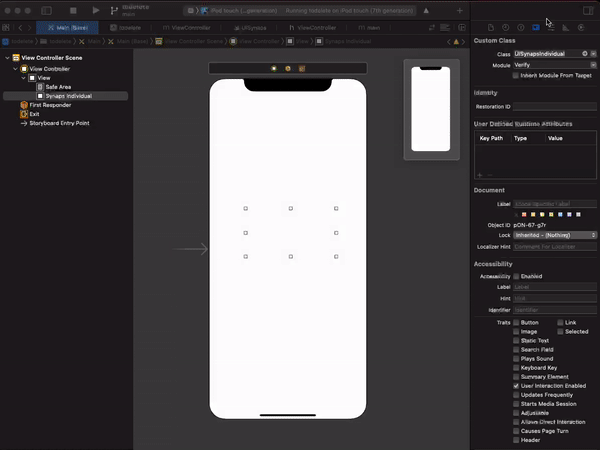
Now you are ready to customize your UISynapsIndividual or UISynapsCorporate from the Attributes Inspector
of Interface Builder.
Attributes
- Name
lang
- Type
- string (optional)
- Description
User language preference
en - English
fr - French
de - German
es - Spanish
it - Italian
ja - Japanese
ko - Korean
pt - Portuguese
ro - Romanian
ru - Russian
tr - Turkish
vi - Vietnamese
zh-CN - Simplified Chinese
zh-TW - Traditional Chinese
- Name
tier
- Type
- string
- Description
Tier ID
3. How to launch it
Make sure to replace your SESSION_ID
by a session_id
fetch from your
backend API.
import SynapsVeriy
import AVFoundation
class ViewController: UIViewController {
@IBOutlet var synaps : UISynapsIndividual!
override func viewDidLoad() {
super.viewDidLoad()
...
}
func yourSessionHandler() {
switch AVCaptureDevice.authorizationStatus(for: .video) {
case .authorized:
synaps.sessionId = SESSION_ID
case .notDetermined:
AVCaptureDevice.requestAccess(for: .video) { granted in
if granted {
self.synaps.sessionId = SESSION_ID
}
}
...
}
}
Now you are ready to listen Synaps event by listening the event Value Changed
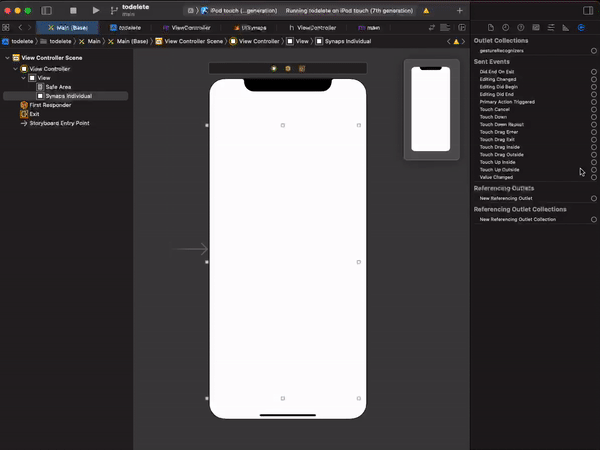
Now you are ready to listen Synaps event by listening the event Value Changed
@IBAction func onStatusChanged(sender: UISynapsIndividual) {
if self.synaps.status == "ready" {
...
} else if self.synaps.status == "finished" {
...
}
}
Android
Add package
To add the Verify SDK to your Android project, follow these steps:
Step 1. Add the JitPack repository to your build.gradle(.kts) file or settings.gradle(.kts)
Gradle
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Step 2. Add the dependency
Gradle
dependencies {
...
implementation("com.github.synaps-io:verify-android-sdk:0.5.0")
}
The Basics
By default, when opening the Verify sdk screen, Camera and NFC permissions will be requested if they have not already been granted. You are free to make the request before opening Verify to integrate it into your user experience. More information about requesting permission in the Android documentation
Compose
Verify SDK provide a simple way to use our service with Android Compose. Just call the VerifyView composable function. A session ID is required.
Kotlin Usage:
Kotlin
VerifyView(
sessionId = "...",
lang = VerifyLang.FRENCH,
tier = "...",
onReady = {
...
},
onFinish = {
...
}
)
Attributes list
Name | Type | Default | Required | Description |
---|---|---|---|---|
sessionId | string | '' | Yes | Session can be referred as a customer verification session. More info |
lang | VerifyLang | VerifyLang.ENGLISH | No | Event listener called on every open/close action |
tier | string | null | No | Tier is a simply way to divide your workflow into small pieces. It is very useful when you offer different features based on the verification level of your customer. More info |
onReady | () => Void | null | No | Event listener called when the page is fully loaded |
onFinished | () => Void | null | No | Event listener called when the user finished verification |
Activity (view legacy)
If you're less familiar with compose, you can use our service by calling an Android Activity.
First, you need to add VerifyActivity
in your Android Manifest:
xml
<activity
android:name="io.synaps.sdk.activity.VerifyActivity"
android:screenOrientation="portrait"
android:exported="true"
tools:ignore="LockedOrientationActivity"/>
Second, To launch the Verify activity, you need to create an intent
and transmit a list of attibutes. Some parameters are optional :
Attributes list
Name | Type | Default | Required | Description |
---|---|---|---|---|
sessionId | string | '' | Yes | Session can be referred as a customer verification session. More info |
lang | VerifyLang | VerifyLang.ENGLISH | No | Event listener called on every open/close action |
tier | string | null | No | Tier is a simply way to divide your workflow into small pieces. It is very useful when you offer different features based on the verification level of your customer. More info |
Kotlin Usage:
Kotlin
val intent = Intent(this, VerifyActivity::class.java)
intent.putExtra("sessionId", "...")
intent.putExtra("lang", VerifyLang.ENGLISH)
intent.putExtra("tier", "...")
startActivity(intent)
Android Jetpack Navigation
You can also use Android Jetpack Navigation instead of basic Intent. Please add in your navigation graph:
xml
<argument
android:name="sessionId"
app:argType="string" />
<argument
android:name="lang"
android:defaultValue="ENGLISH"
app:argType="io.synaps.sdk.core.VerifyLang" />
<argument
android:name="tier"
app:argType="string"/>
Languages available
en - English
fr - French
de - German
es - Spanish
it - Italian
ja - Japanese
ko - Korean
pt - Portuguese
ro - Romanian
ru - Russian
tr - Turkish
vi - Vietnamese
zh-CN - Simplified Chinese
zh-TW - Traditional Chinese